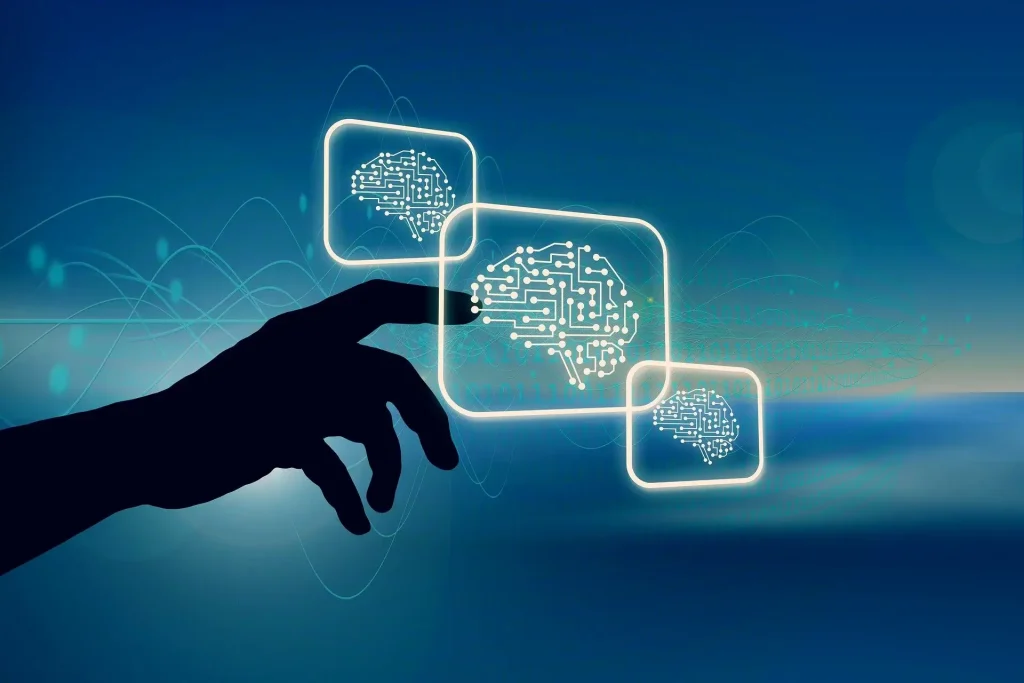
If you’re excited about artificial intelligence and especially AI development, you know that Python is an alpha programming language for this purpose. Its qualities like simplicity, readability, and extensive libraries make it a preferred choice not only for beginners but for senior developers as well. In this article, we will guide you through the basics of implementing simple AI in Python, offering valuable insights and practical tips to enhance your learning journey.
Introduction to Python and Its Role in AI Development
Python is indeed a premiere choice for AI development on any seniority or project complexity level. It’s employed in small startups and large enterprises, as well as by developers of different seniority levels – all thanks to this programming language’s traits.
Python’s syntax is straightforward, making it easy to learn and use, especially for beginners. Its extensive libraries and frameworks, such as TensorFlow, Keras, and PyTorch, offer ready-to-use components for building AI models. This flexibility allows developers to focus on solving problems rather than getting bogged down by complex coding challenges.
Getting Started with Simple AI in Python
Greatness doesn’t equal daunting: simple AI concepts can be implemented with minimal code, encouraging beginners to dive in and start experimenting. Let’s explore some basic AI algorithms and how they can be applied using Python.
Basic AI Algorithms in Python
One of the simplest AI algorithms to understand is the Linear Regression. It’s a statistical method that models the relationship between a dependent variable and one or more independent variables. Here’s a basic example of how to implement linear regression in Python using the popular `scikit-learn` library:
```python
from sklearn.linear_model import LinearRegression
import numpy as np
Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1, 3, 2, 5, 4])
Create and train the model
model = LinearRegression().fit(X, y)
Make predictions
predictions = model.predict(np.array([[6]]))
print(predictions) # Output: [4.8]
```
Another fundamental algorithm is K-Nearest Neighbors (KNN), which can be used for both classification and regression problems. It works by finding the ‘k’ closest data points in the training set and making predictions based on their values.
Python Libraries for AI Development
Python’s rich ecosystem of libraries makes AI development accessible and efficient. Here are some of the most commonly used libraries:
- NumPy: Essential for numerical computations and handling arrays.
- Pandas: Perfect for data manipulation and analysis.
- Matplotlib and Seaborn: Great for data visualization.
- scikit-learn: Provides simple and efficient tools for data mining and data analysis.
- TensorFlow and Keras: Used for developing and training deep learning models.
These libraries offer a robust foundation for creating and experimenting with AI models, making Python an indispensable tool for any AI developer.
Real-world Applications of Python in AI
Python’s versatility and power have led to its widespread adoption in various industries. Here are some real-world applications of Python in AI that highlight its impact and utility:
Healthcare
In healthcare, Python is used to develop models that can predict diseases, analyze medical images, and even suggest treatment plans. For instance, the application of AI in diagnosing diseases from MRI scans has been a game-changer, improving the accuracy and speed of diagnosis.
Finance
The finance industry leverages Python for predictive analytics, fraud detection, and algorithmic trading. AI algorithms can analyze vast amounts of financial data to identify trends, make predictions, and automate trading decisions, contributing to more efficient and profitable operations.
Retail
Retailers use Python-based AI solutions to optimize inventory, recommend products, and personalize customer experiences. AI models analyze consumer behavior and preferences, enabling businesses to enhance their marketing strategies and increase sales. These examples demonstrate Python’s significant role in accelerating AI development and its profound impact across different sectors.
Tips for Mastering Python for AI
To become proficient in using Python for AI development, consider the following tips and resources:
Learning Resources
There are numerous online platforms and courses that can help you learn Python and AI. Websites like Coursera, edX, and Udacity offer specialized courses in AI and machine learning. Additionally, YouTube channels and blogs provide valuable tutorials and insights.
Practice Coding
Consistent practice is crucial for mastering Python and AI. Participate in coding challenges on platforms like LeetCode, HackerRank, and Kaggle. Working on real-world projects and contributing to open-source projects can also enhance your skills and understanding.
Stay Updated
The field of AI is continually evolving. Stay informed about the latest trends, technologies, and best practices by following industry news, joining AI communities, and attending conferences and webinars.
Conclusion and Next Steps
Python’s significance in AI development is the same as C# role in game dev or HTML in web development. Its simplicity, combined with its powerful libraries and frameworks, makes it an ideal choice for creating intelligent systems. Like anything truly revolutionary, Python evolves as tech stack; if you keep your hand on the pulse, your personal development as an engineer will come naturally with Python’s advancement too.