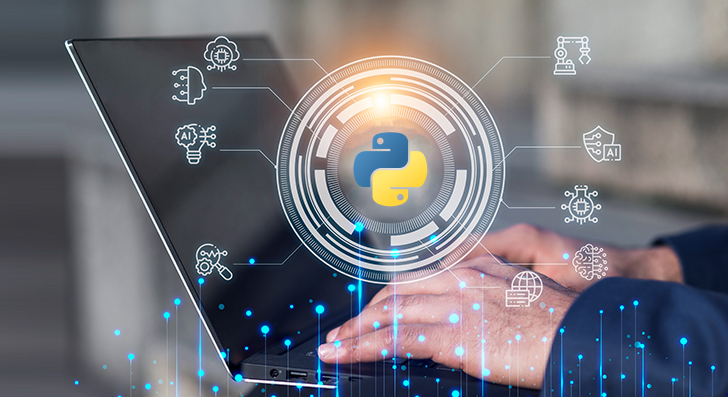
Wherever you go in terms of AI development, it’s almost always Python at a front door. Developers and CTOs love it for a number of qualities; Python exceeds R, Java, C++ and even historical LISP in terms of popularity of AI projects, standing out as an absolutely preferred choice. But why is this programming language so significant in the realm of AI? Let’s try and find out.
The Significance of Python in AI Development
Python has emerged as the go-to language for AI and machine learning (ML) for several compelling reasons:
Simplicity and Readability: Python’s syntax is clear, concise, and intuitive, which makes it easier for developers to write, read, and understand code. This simplicity reduces the learning curve for beginners and helps experienced programmers avoid common coding errors.
Extensive Libraries and Frameworks: Python proudly showcases a rich and effective ecosystem of libraries and frameworks such as TensorFlow, Keras, and PyTorch. These tools provide pre-built modules and functions that simplify complex AI tasks like machine learning, deep learning, and data analysis. With these resources, developers can focus more on innovation rather than reinventing the wheel.
Community Support: Python has one of the largest and most active communities in the programming world. This vibrant community offers a wealth of resources, including comprehensive documentation, tutorials, and forums. Developers can easily find solutions to their problems, share knowledge, and collaborate on projects, speeding up the development process and improving code quality.
Versatility: Python is incredibly versatile as it supports various programming paradigms, from object-oriented and procedural programming to functional programming. This flexibility allows it to be adaptable for different AI projects, whether it’s for data manipulation, AI model development, or automation. Python’s versatility makes it an ideal choice for a wide range of applications, from simple scripts to complex machine learning algorithms.
Overview of Popular AI Programs Developed Using Python
The grasp of greatness shows best on a tangible example. Let’s review a couple of different successful AI programs based on Python:
TensorFlow: Developed by Google Brain, TensorFlow is an open-source framework that facilitates deep learning research and production. It provides a comprehensive ecosystem of tools, libraries, and community resources that empowers researchers and developers to build and deploy machine learning models efficiently.
Keras: An accessible and user-friendly neural networks API, Keras runs on top of TensorFlow, allowing for rapid experimentation. It is designed to enable quick and easy prototyping, supporting both convolutional and recurrent networks, as well as combinations of the two.
OpenAI’s GPT-3: One of the most advanced language models, GPT-3, leverages Python for natural language processing tasks. With 175 billion parameters, it can generate human-like text, translate languages, compose poetry, and even write code, making it a versatile tool for various applications.
Scikit-Learn: A powerful library for machine learning in Python, Scikit-Learn supports various algorithms for data mining and data analysis. It is built on NumPy, SciPy, and Matplotlib, and offers tools for classification, regression, clustering, and dimensionality reduction, making it a go-to resource for data scientists and analysts.
Step-by-Step Guide to Creating a Simple AI Program in Python
Let’s walk through creating a simple AI program in Python. We’ll build a basic neural network using TensorFlow and Keras to recognize handwritten digits from the MNIST dataset.
Step 1: Installing Required Libraries
First, ensure you have Python installed. Then, install TensorFlow and Keras using pip:
```bash
pip install tensorflow keras
```
Step 2: Importing Libraries
Next, import the required libraries:
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.utils import to_categorical
```
Step 3: Loading and Preprocessing Data
Load and preprocess the MNIST dataset:
```python
Load dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
Normalize pixel values
x_train, x_test = x_train / 255.0, x_test / 255.0
One-hot encode labels
y_train, y_test = to_categorical(y_train), to_categorical(y_test)
```
Step 4: Building the Model
Create a simple neural network model:
```python
Initialize the model
model = Sequential()
Add layers
model.add(Flatten(input_shape=(28, 28)))
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
```
Step 5: Training the Model
Train the model on the training data:
``python
model.fit(x_train, y_train, epochs=5, batch_size=32, validation_split=0.2)
```
Step 6: Evaluating the Model
Finally, evaluate the model on the test data:
```python
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
Congratulations! You’ve just created a simple AI program in Python. This example demonstrates how accessible AI coding in Python can be, even for beginners.
Advice for Python Developers Looking to Enhance Their AI Skills
Want to strengthen the Python muscle? Here are some workout tips.
- Stay Updated: The field of AI is rapidly evolving. Follow industry news, research papers, and updates on AI frameworks to stay abreast of the latest advancements and trends and ensure you’re always in the know.
- Hands-On Practice: Regularly engage with coding exercises and real-world projects to apply your knowledge. Practice is crucial in mastering AI concepts and techniques, so take on various projects to build a well-rounded skill set.
- Online Courses and Tutorials: Platforms like Coursera, Udacity, and edX offer comprehensive courses on AI and machine learning. These courses often include interactive modules, hands-on labs, and expert instruction, which can significantly enhance your understanding.
- Participate in Competitions: Engage in data science competitions on platforms like Kaggle to test your skills against peers. Competitions provide a practical setting to apply your learning, solve complex problems, and even earn recognition in the AI community.
- Join Communities: Become a member in AI and Python communities on forums like Stack Overflow and GitHub to learn from and contribute to collective knowledge. Engaging with these communities can provide support, mentorship, and collaboration opportunities, enriching your learning journey.
Conclusion
The advantages of Python in terms of AI coding will long overweight any other language’s list of pros. With time, it doesn’t age – on the contrary, the community keeps coming up with robust tools that help AI projects thrive.
If you are ready to start your journey into AI coding in Python, our advise is simply to start. Dive in, experiment, and continue learning. The future of AI awaits your contribution. Happy coding!