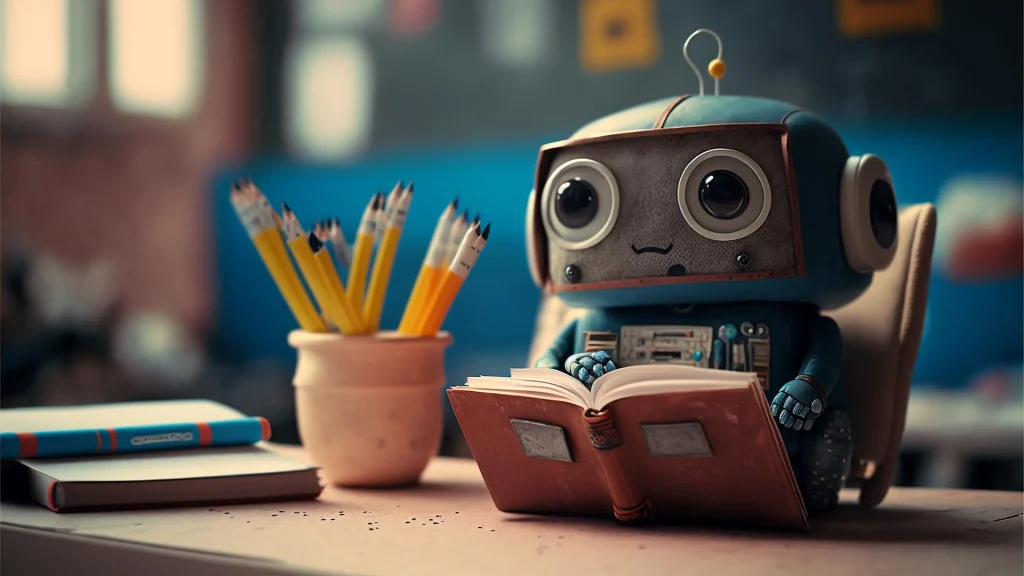
We know how much AI engineers value Python: it drastically stands out among other possible tech stacks for artificial intelligence development, shining with versatility, agility and simple syntax. Let’s explore the symbiotic relationship between AI and Python, diving into the essentials of Python programming for AI, key libraries, practical implementation, and best practices to elevate your skills. We will combine the general knowledge with exclusive tips we’ve come across in terms of using Python for AI.
Introduction to AI and Python
Artificial Intelligence has captivated the imagination of many, promising innovations that range from autonomous vehicles to personalized healthcare. As AI continues to evolve, one language stands out for its simplicity and efficacy in AI development — Python. But why Python? Its readability, extensive library support, and strong community presence have made it indispensable for AI programmers. For tech enthusiasts, understanding Python’s role in AI can open doors to limitless opportunities in this dynamic field.
The demand for AI solutions has led to a significant surge in the popularity of Python. Its straightforward syntax and robust capabilities make it accessible for beginners while powerful enough for experts working on complex AI models. As you embark on this journey, you’ll discover how Python simplifies the intricacies of AI programming, making it a preferred choice for both novice developers and industry veterans.
In this article, we’ll cover the basics of Python for AI, introduce essential AI libraries, provide a practical guide to AI programming, and share resources and best practices to help you excel. By the end, you’ll be well-equipped to delve deeper into the exciting world of AI with Python.
The Basics of Python for AI
There are some foundational concepts of Python – the basics shaping the building blocks of more advanced AI applications and ensuring a smooth learning curve.
Understanding Data Types
Python offers a variety of data types that you’ll often use in AI programming, such as integers, floats, strings, and lists. Knowing how to utilize these basic data types effectively is essential. For instance, data manipulation is a core aspect of AI, and Python’s list and dictionary types provide flexible ways to store and manage data.
Control Structures and Functions
Control structures like loops and conditional statements enable the creation of dynamic and responsive AI programs. Functions allow you to encapsulate code logic, making your program modular and easier to manage. Understanding how to define and call functions will enable you to build sophisticated AI algorithms with ease.
Introduction to Object-Oriented Programming
AI models are often represented as objects. Python’s object-oriented programming (OOP) paradigm allows you to create classes and objects that can encapsulate data and behavior. This approach is particularly useful in AI programming, where models often need to maintain state and perform complex operations.
Essential AI Libraries in Python
One of Python’s greatest strengths lies in its extensive ecosystem of libraries tailored for AI development. Here, we’ll explore three key libraries that are instrumental in AI programming with Python.
TensorFlow
Developed by Google, TensorFlow is a powerful library for numerical computation and large-scale machine learning. It offers a flexible platform for building and deploying AI models, from simple linear regressions to cutting-edge neural networks. TensorFlow’s compatibility with various hardware accelerators makes it a staple for AI practitioners.
Keras
Keras is an open-source neural network library that operates as a high-level API for TensorFlow. It simplifies the process of building and training deep learning models, offering intuitive and user-friendly abstractions for neural networks. Because of straightforward interface, Keras is ideal for beginners while providing the depth needed for advanced research.
Scikit-learn
Scikit-learn is a comprehensive library for machine learning in Python. It includes tools for classification, regression, clustering, and dimensionality reduction. Scikit-learn’s simplicity and efficiency make it an excellent choice for implementing machine learning algorithms, from basic to complex models.
Practical AI Programming with Python
Learning the theory behind AI is important, but applying that knowledge through practical coding is where true mastery begins. Let’s walk through a simple AI program using Python, highlighting key concepts and techniques.
Setting Up Your Environment
First, ensure you have Python installed on your system along with the necessary libraries. You can use `pip` to install TensorFlow, Keras, and Scikit-learn:
```bash
pip install tensorflow keras scikit-learn
```
Building a Simple Neural Network
Here, we’ll create a simple neural network using Keras to classify handwritten digits from the famous MNIST dataset. This example demonstrates how to implement a basic AI model and understand the workflow of AI programming.
```python
import numpy as np
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense, Flatten
from keras.utils import to_categorical
Load dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
Preprocess the data
x_train = x_train / 255.0
x_test = x_test / 255.0
y_train = to_categorical(y_train)
y_test = to_categorical(y_test)
Build the model
model = Sequential([
Flatten(input_shape=(28, 28)),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
Train the model
model.fit(x_train, y_train, epochs=5, batch_size=32)
Evaluate the model
loss, accuracy = model.evaluate(x_test, y_test)
print(f'Accuracy: {accuracy * 100:.2f}%')
```
Understanding the Code
- Data Loading and Preprocessing: The MNIST dataset is loaded and normalized to ensure the pixel values are between 0 and 1. Labels are converted to categorical format.
- Model Construction: A sequential model is built with an input layer, a hidden layer (with ReLU activation), and an output layer (with Softmax activation).
- Model Compilation: The model is compiled using the Adam optimizer and categorical cross-entropy loss function.
- Training and Evaluation: The model is trained on the training set and evaluated on the test set, providing an accuracy metric.
Best Practices and Resources for Learning AI Programming in Python
Mastering AI programming with Python requires dedication, practice, and access to quality resources. Here are some tips and tools to help you on your journey.
Continuous Learning
AI is a rapidly evolving field. Stay updated with the latest trends and advancements by following industry blogs, attending webinars, and enrolling in online courses. Platforms like Coursera, Udacity, and edX offer specialized AI and machine learning courses.
Practice Through Projects
Hands-on experience is invaluable. Work on real-world projects that interest you, such as image classification, natural language processing, or predictive analytics. Websites like Kaggle provide datasets and competitions to test and improve your skills.
Join Communities
Engage with the AI and Python programming communities. Forums like Stack Overflow, Reddit, and specialized groups on LinkedIn offer support, knowledge sharing, and networking opportunities. Being part of a community can accelerate your learning and provide valuable insights.
Conclusion and Next Steps
Python has firmly established itself as a cornerstone in the realm of AI programming. Its simplicity, combined with powerful libraries, makes it an ideal choice for both beginners and experts. If you’d like to deepen your expertise, start by implementing more complex models and contributing to open-source AI projects. This practice will pay off with broad feedback from the Python community, while educating yourself and keeping a hand on the pulse of Python news will retain your value as a potent engineer.