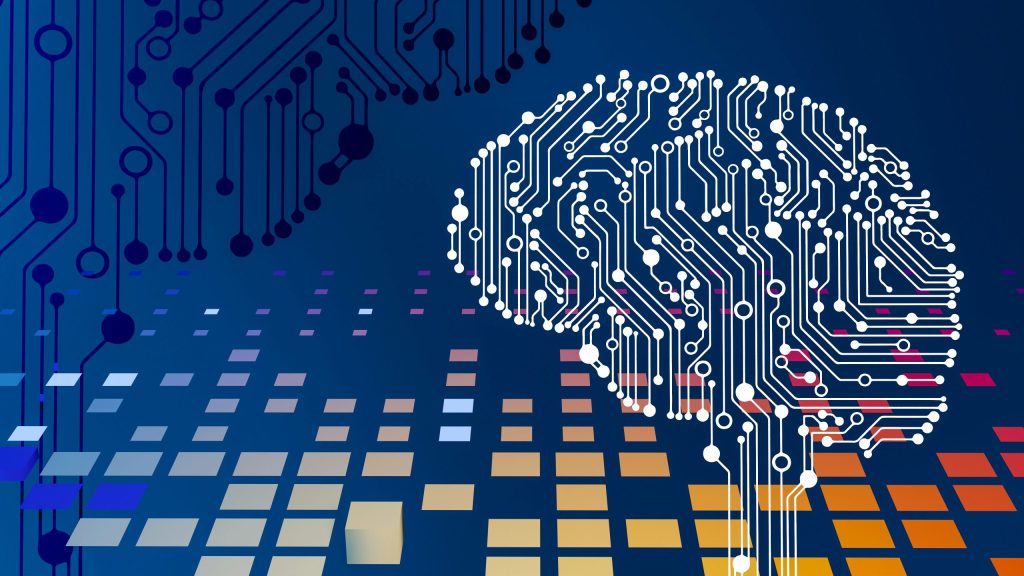
Tic Tac Toe, also known as Noughts and Crosses, is a simple yet profound game coming from Ancient times. Its perfect blend of strategy, logic, and simplicity makes it not just historical but a referent of many patterns. For Python developers, AI enthusiasts, and tech learners, Tic Tac Toe serves as an excellent entry point into the world of artificial intelligence and game theory. In fact, about 75% of introductory AI courses include Tic Tac Toe as a case study for teaching basic principles of game theory and decision-making algorithms.
Let’s then try and walk through implementing a Tic Tac Toe game in Python, explore AI strategies like the minimax algorithm, and learn how to create an unbeatable AI opponent.
The Significance of Tic Tac Toe in AI and Game Theory
Why Tic Tac Toe?
Tic Tac Toe used to be just a game from everyone’s early childhood, while simultaneously, it’s a gateway into the principles of game theory and artificial intelligence. Its straightforward rules make it an ideal platform for understanding complex algorithms without getting bogged down by intricate mechanics. For developers, mastering Tic Tac Toe AI in Python is a stepping stone to more complicated projects like chess AI or even real-time strategy games.
Historical Context
The study of Tic Tac Toe dates back to the early days of computer science. Researchers used it as a model to explore algorithmic strategies and decision-making processes. In game theory, it serves as a classic example of a zero-sum game where one player’s gain is another player’s loss. This makes it an excellent study subject for minimax algorithms and other decision-making models.
Relevance Today
In today’s tech landscape, AI is everywhere — from self-driving cars to recommendation systems. Starting with Tic Tac Toe allows developers to grasp the fundamentals of AI and gradually progress to more complex applications. Understanding how to implement strategies in a simple game can provide valuable insights into tackling larger, real-world problems.
Basics of Implementing Tic Tac Toe in Python
Setting Up the Game Board
The first step in creating a Tic Tac Toe game is setting up the game board. In Python, this can be represented using a 2D list. A 3×3 grid is typical, where each cell can be empty, contain an ‘X,’ or contain an ‘O.’
```python
board = [[' ' for _ in range(3)] for _ in range(3)]
```
This initializes a 3×3 grid filled with blank spaces. Each move will update this board, making it easy to check for wins or draws.
Implementing Game Logic
Next, let’s implement the core game logic. This includes functions to make a move, check for a win, and determine if the board is full.
```python
def make_move(board, row, col, player):
if board[row][col] == ' ':
board[row][col] = player
return True
return False
def check_win(board, player):
for row in board:
if all([cell == player for cell in row]):
return True
for col in range(3):
if all([board[row][col] == player for row in range(3)]):
return True
if all([board[i][i] == player for i in range(3)]) or all([board[i][2-i] == player for i in range(3)]):
return True
return False
def is_board_full(board):
return all([cell != ' ' for row in board for cell in row])
```
These functions handle the essential operations of making moves, checking for wins, and determining if the board is full.
User Interface
A simple text-based interface can suffice for our Tic Tac Toe game. Here’s a basic function to print the board:
```python
def print_board(board):
for row in board:
print('|'.join(row))
print('-' * 5)
```
This prints the board in a readable format, making it easy for players to see the current state of the game.
Exploring AI Strategies: The Minimax Algorithm
What is Minimax?
The minimax algorithm is a decision-making algorithm used in game theory and AI. It aims to minimize the possible loss in a worst-case scenario while maximizing potential gain. This makes it perfect for two-player turn-based games like Tic Tac Toe and chess AI Python applications.
How Minimax Works
Minimax operates by exploring all possible moves from a given state, assuming the opponent will always make the best possible counter-move. It then selects the move that maximizes the player’s minimum guaranteed outcome.
Implementing Minimax in Python
Here’s a simplified version of the minimax algorithm for Tic Tac Toe:
```python
def minimax(board, depth, is_maximizing):
if check_win(board, 'X'):
return 1
elif check_win(board, 'O'):
return -1
elif is_board_full(board):
return 0
if is_maximizing:
best_score = float('-inf')
for row in range(3):
for col in range(3):
if board[row][col] == ' ':
board[row][col] = 'X'
score = minimax(board, depth + 1, False)
board[row][col] = ' '
best_score = max(score, best_score)
return best_score
else:
best_score = float('inf')
for row in range(3):
for col in range(3):
if board[row][col] == ' ':
board[row][col] = 'O'
score = minimax(board, depth + 1, True)
board[row][col] = ' '
best_score = min(score, best_score)
return best_score
```
This function evaluates all possible moves and selects the best one based on the minimax principle.
Integrating Minimax into the Python Tic Tac Toe Game
Choosing the Best Move
To integrate minimax into our game, we need a function to choose the best move for the AI:
```python
def best_move(board):
best_score = float('-inf')
move = None
for row in range(3):
for col in range(3):
if board[row][col] == ' ':
board[row][col] = 'X'
score = minimax(board, 0, False)
board[row][col] = ' '
if score > best_score:
best_score = score
move = (row, col)
return move
```
This function iterates over all possible moves, applies the minimax algorithm, and returns the optimal move.
Playing Against the AI
Finally, let’s add functionality for the player to compete against our AI:
```python
def play_game():
board = [[' ' for _ in range(3)] for _ in range(3)]
while True:
print_board(board)
row, col = map(int, input("Enter your move (row and column): ").split())
if make_move(board, row, col, 'O'):
if check_win(board, 'O'):
print("You win!")
break
if is_board_full(board):
print("Draw!")
break
ai_move = best_move(board)
make_move(board, ai_move[0], ai_move[1], 'X')
if check_win(board, 'X'):
print("AI wins!")
break
if is_board_full(board):
print("Draw!")
break
else:
print("Invalid move! Try again.")
```
This function allows the player to make moves against the AI, providing a complete gameplay experience.
Analysis of the Implementation
Strengths
Our Tic Tac Toe AI in Python is robust and unbeatable. It leverages the minimax algorithm to make optimal moves, ensuring the AI cannot lose. This makes it an excellent educational tool for understanding game theory and AI.
Weaknesses
One limitation of this implementation is its computational efficiency. The minimax algorithm can be slow for more complex games like chess. However, optimizations such as alpha-beta pruning can mitigate this issue, making it more suitable for larger games.
Potential Improvements
Future enhancements could include adding a graphical user interface (GUI) for a more engaging user experience. Additionally, implementing advanced strategies to handle more complex games like chess AI Python could be a logical next step.
Conclusion
Many genius things are based on simplicity. Tic Tac Toe is one such basis; it may seem uncomplicated, but its application in AI and game theory goes beyond simple mechanics. By implementing a Tic Tac Toe game in Python and integrating the minimax algorithm, the game development engineers can create an unbeatable AI opponent. This project not only provides a solid foundation in AI principles but also opens the door to more complex projects like how to make a chess AI in Python. For those eager to take their gamedev expertise deeper, experimenting with enhancements like GUIs or tackling more intricate games can be the next exciting challenge.